Learn TypeScript With Me in 14 Days Day 1
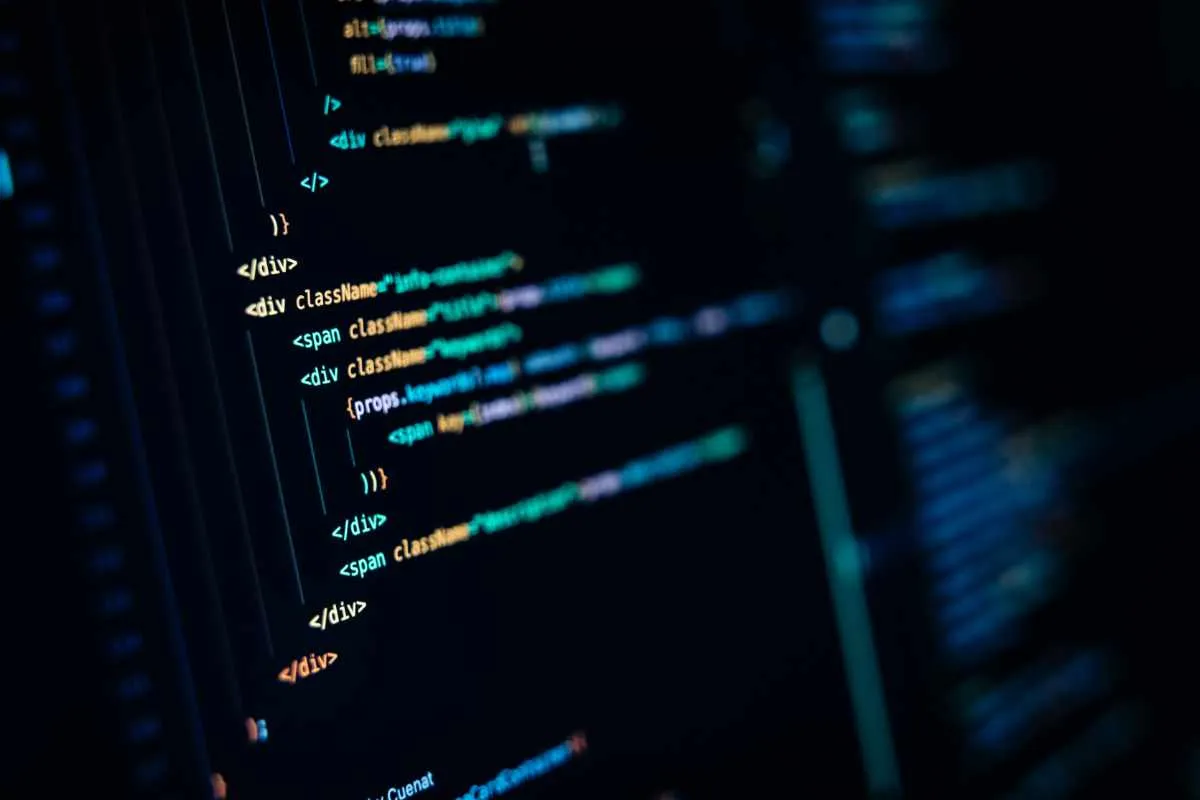
Personal Journey: Why I Delayed Learning TypeScript
When I first started as a junior developer, my relationship with JavaScript was, to put it lightly, complicated. As someone who preferred writing backend code, I spent most of my time in the comfort zone of PHP, focusing on structured, server-side logic. When I was thrust into front-end development, it felt like being dropped in a foreign land. My understanding of JavaScript concepts was basic at best, and I often struggled with its quirks like asynchronous code, closures, and the lack of strict typing.
The transition from PHP to JavaScript was not an easy one for me. While PHP had a more structured, server-side logic that I understood, JavaScript’s dynamic and sometimes unpredictable nature often left me frustrated. For a long time, I stuck to basic jQuery to get things done, but I always felt like I was patching solutions rather than deeply understanding the language.
As I slowly learned more about JavaScript, things began to make more sense, but by then I had developed a sort of resistance to moving beyond it—especially when I heard about TypeScript. I felt like I had just finally grasped JavaScript. Why complicate things again by introducing a superset language like TypeScript?
Why TypeScript Now? Over time, as I took on larger and more complex JavaScript projects, the shortcomings of dynamic typing became apparent. Simple type-related errors that could have been caught early caused bugs that took hours to troubleshoot. Watching my colleagues adopt TypeScript and seeing the benefits they reaped, I knew it was time to stop delaying and dive in. I realized that TypeScript could actually help me write better, more reliable code—and that it wasn’t something to be afraid of but rather a tool to help avoid the very issues I struggled with in JavaScript.
So, here we are! Over the next 14 days, we’ll learn TypeScript together, and I’ll share my insights, frustrations, and breakthroughs with you in real-time.
What is TypeScript?
TypeScript is a superset of JavaScript developed by Microsoft that adds static typing to the language. It allows you to catch potential bugs at compile time rather than runtime, making your code more reliable and maintainable.
Key Features of TypeScript:
- Static Typing: Helps catch type-related errors early.
- Optional Types: You can add types incrementally to existing JavaScript code.
- Modern JavaScript Features: TypeScript supports the latest ECMAScript standards and transpiles down to compatible JavaScript.
- Great Tooling: TypeScript provides better autocompletion, type checking, and refactoring capabilities in editors like VSCode.
Why TypeScript?
- You avoid a lot of runtime errors by catching issues early.
- It enhances code readability and makes the codebase easier to maintain.
- As projects scale, TypeScript reduces the risk of type errors, which becomes invaluable for large teams and complex applications.
Project Setup: Task Manager
Throughout the next 14 days, we will be building a simple Task Manager app where you can create, update, and manage tasks. By the end of the series, you’ll have a fully working app written in TypeScript.
Step 1: Setting Up TypeScript
1. Install Node.js and npm
If you haven’t already, you need to install Node.js, which comes with npm (Node Package Manager). This will allow you to install TypeScript and other development dependencies.
- Download Node.js (choose the LTS version)
- Verify the installation by running these commands in your terminal:
node -v npm -v
2. Install TypeScript
Once Node.js is installed, you can install TypeScript globally on your system using npm. Open your terminal and run:
npm install -g typescript
You can verify the installation by running:
tsc -v
This command will show you the installed version of TypeScript.
3. Initialize a TypeScript Project
Now, let’s initialize a new TypeScript project. Create a folder for your project and navigate into it:
mkdir task-manager
cd task-manager
Inside this folder, initialize a new TypeScript project:
tsc --init
This creates a tsconfig.json file, which contains all the configuration options for TypeScript. In the compilerOptions options, set the outDir
to ./dist You can leave the remaining settings for now.
A Brief Overview of the tsconfig.json file
The tsconfig.json file is the core configuration file for your TypeScript project. It controls how TypeScript compiles the code. Here are a few important settings:
- “target”: Specifies the JavaScript version that TypeScript should compile down to. By default, it’s set to es5, but you can change it to es6 or newer if needed.
- “outDir”: This specifies where the compiled JavaScript files should go. We set it to “dist” in our case.
- “rootDir”: This tells TypeScript where the source files are located. We’ll be working from the src folder.
You can edit these settings later to match the needs of your project.
4. Setting up the Project Directory
Let’s organize our project folder. In the root folder of your project, create two directories:
mkdir src dist
- src: This is where we will write our TypeScript code.
- dist: This is where the compiled JavaScript code will be output.
Step 2: Writing Your First TypeScript Program
Now let’s create our first TypeScript file inside the src
folder.
- Create a File: Inside the src folder, create a file called index.ts.
touch src/index.ts
- Add a Simple Program: Open index.ts in your editor (e.g., VSCode), and add the following code:
let greeting: string = 'Hello, TypeScript!';
console.log(greeting);
Here, you are explicitly typing the variable greeting as a string. If you try to assign a non-string value to the greeting variable, TypeScript will throw an error at compile time, catching the issue before you even run the code.
Step 3: Compiling TypeScript
To convert the TypeScript code into JavaScript, we need to compile it. Run the following command in your terminal:
tsc
This command compiles all .ts
files in the src
folder based on the configuration in tsconfig.json
. The compiled JavaScript file will be placed in the dist
folder. You should see a new file at dist/index.js
.
var greeting = 'Hello, TypeScript!';
console.log(greeting);
Now, you can run the generated JavaScript code using Node.js:
node dist/index.js
You should see the output:
Hello, TypeScript!
Let’s try another example by creating a simple function that takes two numbers as arguments and returns their sum. We will use TypeScript to enforce that both arguments are numbers.
let firstNumber: number = 5;
let secondNumber: number = 10;
let result: number = firstNumber + secondNumber;
console.log(result);
If you compile the above and run it, you should get the output 15.
Congratulations! You’ve just written and compiled your first TypeScript program.
What’s Next?
Today was all about getting our environment set up and writing our first TypeScript program. Tomorrow, we’ll dive deeper into TypeScript by exploring basic types. We’ll use this knowledge to start defining types for the tasks in our project and lay the groundwork for our Task Manager app.
I hope today’s session was fun and informative! Tomorrow, we’ll start diving deeper into TypeScript’s powerful type system. Stay tuned for Day 2: Basic Types.
Let me know if you have any questions or if you had any issues getting everything set up by contacting me at steve [at] stephenpopoola.uk.