An Introduction to Attributes in PHP
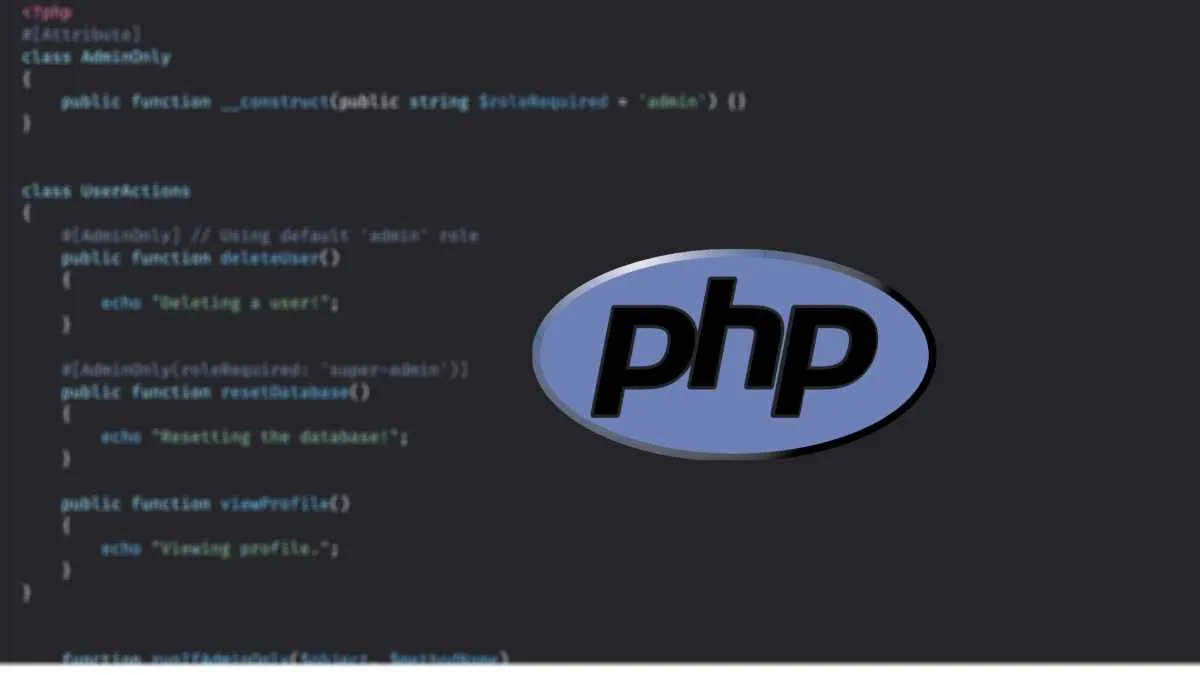
An Introduction to Attributes in PHP
In PHP 8, a new feature called Attributes was introduced. Attributes are like labels or tags that you can add to parts of your code (like classes, properties, and methods) to give them extra information. Think of attributes as a way to add metadata that your program can read and use to control how things behave.
Before PHP 8, if you wanted to add extra information to parts of your code, you often used comments (like PHPDoc) to document things or store data. However, comments aren’t directly readable by the code—they’re just for humans to read. Attributes, on the other hand, allow you to store data that PHP can read and use while the code is running.
Attributes can make your code:
- Easier to read by putting configuration right where it’s used.
- More organized since metadata is stored alongside the code it describes.
- Dynamic because PHP can directly interact with the attributes at runtime.
How to Use Attributes: A Basic Example
Let’s start with a simple example. Say we want to mark certain functions in our code as requiring authentication. We’ll define an attribute called RequiresAuth.
To create an attribute in PHP, you define a class and use #[Attribute] at the top.
Define the Attribute
<?php
#[Attribute] // This makes the class usable as an attribute
class RequiresAuth
{
public function __construct(public bool $required = true) {}
}
Apply the Attribute
Now that we have the RequiresAuth attribute, let’s apply it to a method that should require authentication.
class AccountController
{
#[RequiresAuth]
public function viewProfile()
{
// Code for viewing profile
}
}
This tells PHP that the viewProfile() method should only be accessed if the user is authenticated.
Attributes add useful, structured data to your code, and while reflection is the tool used to access this data, we can ease into it with practical examples. Think of reflection as a way for PHP to look at itself—its functions, classes, and attributes—while your code is running.
If like me, you are using a PHP Framework like Laravel, you can use attributes to simplify certain parts of your code.
Custom Validation Rules with Attributes
When working with models in Laravel, you often need to validate user data. With attributes, you can add validation rules directly to model properties. This keeps the rules close to the data they apply to.
#[Attribute]
class Validate
{
public function __construct(public string $rule) {}
}
Now, apply the Validate attribute to properties in a model. Let’s add it to a User model:
class User
{
#[Validate('required|email')]
public string $email;
#[Validate('required|min:8')]
public string $password;
}
We can write a function to read these validation rules and apply them dynamically:
function getValidationRules(object $model)
{
$reflection = new ReflectionClass($model);
$rules = [];
foreach ($reflection->getProperties() as $property) {
$attributes = $property->getAttributes(Validate::class);
foreach ($attributes as $attribute) {
$rule = $attribute->newInstance()->rule;
$rules[$property->getName()] = $rule;
}
}
return $rules;
}
// Usage
$user = new User();
$rules = getValidationRules($user);
// Now $rules can be used in Laravel's Validator
Attributes rely heavily on Reflection. If you have not come across or used reflection, think of reflection as a way for PHP to look at itself—its functions, classes, and attributes—while your code is running.
What is Reflection?
Reflection allows PHP to examine parts of its own code (classes, properties, methods, etc.) during runtime. In the context of attributes, reflection helps us find and use the data stored in those attributes.
Let’s break this down with a straightforward example.
Setting Up a Basic PHP Attribute
Imagine you’re building a system where some methods should only be used by administrators. You could create an attribute to mark these methods and check for the attribute when the method is called.
<?php
#[Attribute]
class AdminOnly
{
public function __construct(public string $roleRequired = 'admin') {}
}
This AdminOnly attribute has a property $roleRequired, which we can set when applying the attribute.
class UserActions
{
#[AdminOnly] // Using default 'admin' role
public function deleteUser()
{
echo "Deleting a user!";
}
#[AdminOnly(roleRequired: 'super-admin')]
public function resetDatabase()
{
echo "Resetting the database!";
}
public function viewProfile()
{
echo "Viewing profile.";
}
}
In this example, only deleteUser and resetDatabase methods are restricted by the AdminOnly attribute.
Create a Function to Check for the AdminOnly Attribute
function runIfAdminOnly($object, $methodName)
{
$reflectionMethod = new ReflectionMethod($object, $methodName);
$attributes = $reflectionMethod->getAttributes(AdminOnly::class);
if (!empty($attributes)) {
$attribute = $attributes[0]->newInstance();
echo "This method is restricted to role: {$attribute->roleRequired}\n";
} else {
echo "This method has no role restriction.\n";
}
// Call the method if allowed (for demonstration purposes only)
$object->$methodName();
}
// Testing the function
$userActions = new UserActions();
runIfAdminOnly($userActions, 'deleteUser'); // Outputs role restriction
runIfAdminOnly($userActions, 'viewProfile'); // No role restriction
Explanation of Reflection in This Example
- ReflectionMethod($object, $methodName): This line lets PHP inspect the method on the object.
- getAttributes(AdminOnly::class): This gets any AdminOnly attributes applied to the method.
- newInstance(): This method creates an instance of the attribute, so we can access properties like roleRequired.
This code checks for the AdminOnly attribute and then decides whether to run the method based on that.
Adding Metadata to APIs
Imagine you’re building a simple API, and you want to document which methods are accessible via GET and POST requests.
First, you define the attribute like we did earlier:
#[Attribute]
class Route
{
public function __construct(
public string $method = 'GET',
public string $path = '/'
) {}
}
Now we apply the attribute to the methods:
class ApiController
{
#[Route(method: 'GET', path: '/users')]
public function listUsers()
{
echo "Listing users!";
}
#[Route(method: 'POST', path: '/users')]
public function createUser()
{
echo "Creating user!";
}
}
We can now use reflection to check each method’s attributes to see what HTTP methods and paths are supported.
function getApiRoutes($controller)
{
$reflection = new ReflectionClass($controller);
foreach ($reflection->getMethods() as $method) {
$attributes = $method->getAttributes(Route::class);
foreach ($attributes as $attribute) {
$route = $attribute->newInstance();
echo "Method: {$route->method}, Path: {$route->path}\n";
}
}
}
getApiRoutes(new ApiController());
This prints out the supported HTTP methods and paths, useful for API documentation or routing.
If you are a beginner or are not familiar with reflection, here are a few tips to simplify it:
- Start Small: Use reflection to access attributes on simple classes or methods.
- Use Helper Functions: Wrap reflection code in helper functions (like runIfAdminOnly) to keep the main logic easy to understand.
- Focus on Attributes, Not Reflection: When using attributes, think of reflection as a tool that “looks” at attributes rather than as a complex feature. Over time, it will become more familiar.
Conclusion
Attributes in PHP are powerful tools for adding structured metadata to your code, and reflection allows you to access that metadata. By using attributes, you can make your code more organized and efficient. With these examples, you now have a basic understanding of how to work with attributes without needing a framework like Laravel.
Remember, reflection can feel tricky at first, but with practice, it can become a valuable tool in your PHP toolkit!